Permuting a single dimension (column) of a hypercube. More...
#include <permutations.h>
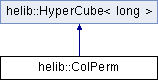
Public Member Functions | |
ColPerm (const CubeSignature &_sig) | |
long | getPermDim () const |
void | setPermDim (long _dim) |
void | makeExplicit (Permut &out) const |
long | getShiftAmounts (Permut &out) const |
void | getBenesShiftAmounts (NTL::Vec< Permut > &out, NTL::Vec< bool > &idID, const NTL::Vec< long > &benesLvls) const |
void | printout (std::ostream &s) |
A test/debugging method. More... | |
![]() | |
HyperCube (const HyperCube &other)=default | |
HyperCube (const CubeSignature &_sig) | |
initialize a HyperCube with a CubeSignature More... | |
HyperCube & | operator= (const HyperCube< long > &other) |
assignment: signatures must be the same More... | |
bool | operator== (const HyperCube< long > &other) const |
equality testing: signatures must be the same More... | |
bool | operator!= (const HyperCube< long > &other) const |
const CubeSignature & | getSig () const |
const ref to signature More... | |
NTL::Vec< long > & | getData () |
const NTL::Vec< long > & | getData () const |
read-only ref to data vector More... | |
long | getSize () const |
total size of cube More... | |
long | getNumDims () const |
number of dimensions More... | |
long | getDim (long d) const |
size of dimension d More... | |
long | getProd (long d) const |
product of sizes of dimensions d, d+1, ... More... | |
long | getProd (long from, long to) const |
product of sizes of dimensions from, from+1, ..., to-1 More... | |
long | getCoord (long i, long d) const |
get coordinate in dimension d of index i More... | |
long | addCoord (long i, long d, long offset) const |
add offset to coordinate in dimension d of index i More... | |
long | numSlices (long d=1) const |
number of slices More... | |
long | sliceSize (long d=1) const |
size of one slice More... | |
long | numCols () const |
number of columns More... | |
long & | at (long i) |
reference to element at position i, with bounds check More... | |
const long & | at (long i) const |
read-only reference to element at position i, with bounds check More... | |
long & | operator[] (long i) |
reference to element at position i, without bounds check More... | |
const long & | operator[] (long i) const |
read-only reference to element at position i, without bounds check More... | |
void | rotate1D (long i, long k) |
rotate k positions along the i'th dimension More... | |
void | shift1D (long i, long k) |
Shift k positions along the i'th dimension with zero fill. More... | |
Detailed Description
Permuting a single dimension (column) of a hypercube.
ColPerm is derived from a HyperCube<long>, and it uses the cube object to store the actual permutation data. The interpretation of this data, however, depends on the data member dim.
The cube is partitioned into columns of size n = getDim(dim): a single column consists of the n entries whose indices i have the same coordinates in all dimensions other than dim. The entries in any such column form a permutation on [0..n).
For a given ColPerm perm, one way to access each column is as follows: for slice_index = [0..perm.getProd(0, dim)) CubeSlice slice(perm, slice_index, dim) for col_index = [0..perm.getProd(dim+1)) getHyperColumn(column, slice, col_index)
Another way is to use the getCoord and addCoord methods.
For example, permuting a 2x3x2 cube along dim=1 (the 2nd dimension), we could have the data std::vector as [ 1 1 0 2 2 0 2 0 1 1 0 2 ]. This means the four columns are permuted by the permutations [ 1 0 2 ] [ 1 2 0 ] [ 2 1 0 ] [ 0 1 2 ]. Written explicitly, we get: [ 2 3 0 5 4 1 10 7 8 9 6 11 ].
Another representation that we provide is by "shift amount": how many slots each element needs to move inside its small permutation. For the example above, this will be: [ 1 -1 0 ] [ 2 -1 -1 ] [ 2 0 -2 ] [ 0 0 0 ] so we write the permutation as [ 1 1 -1 1 0 -2 2 0 0 0 -2 0 ].
Constructor & Destructor Documentation
◆ ColPerm()
|
inlineexplicit |
Member Function Documentation
◆ getBenesShiftAmounts()
void helib::ColPerm::getBenesShiftAmounts | ( | NTL::Vec< Permut > & | out, |
NTL::Vec< bool > & | idID, | ||
const NTL::Vec< long > & | benesLvls | ||
) | const |
Get multiple layers of a Benes permutation network. Returns in out[i][j] the shift amount to move item j in the i'th layer. Also isID[i]=true if the i'th layer is the identity (i.e., contains only 0 shift amounts).
◆ getPermDim()
|
inline |
◆ getShiftAmounts()
long helib::ColPerm::getShiftAmounts | ( | Permut & | out | ) | const |
For each position in the data std::vector, compute how many slots it should be shifted inside its small permutation. Returns zero if all the shift amounts are zero, nonzero values otherwise.
◆ makeExplicit()
void helib::ColPerm::makeExplicit | ( | Permut & | out | ) | const |
◆ printout()
|
inline |
A test/debugging method.
◆ setPermDim()
|
inline |