Implementing polynomials (elements in the ring R_Q) in double-CRT form. More...
#include <DoubleCRT.h>
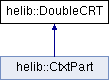
Public Member Functions | |
DoubleCRT (const DoubleCRT &other)=default | |
DoubleCRT (const NTL::ZZX &poly, const Context &_context, const IndexSet &indexSet) | |
Initializing DoubleCRT from a ZZX polynomial. More... | |
DoubleCRT (const zzX &poly, const Context &_context, const IndexSet &indexSet) | |
Same as above, but with zzX's. More... | |
DoubleCRT (const Context &_context, const IndexSet &indexSet) | |
Also specify the IndexSet explicitly. More... | |
DoubleCRT & | operator= (const DoubleCRT &other) |
DoubleCRT & | operator= (const zzX &poly) |
DoubleCRT & | operator= (const NTL::ZZX &poly) |
DoubleCRT & | operator= (const NTL::ZZ &num) |
DoubleCRT & | operator= (const long num) |
long | getOneRow (NTL::Vec< long > &row, long idx, bool positive=false) const |
Get one row of a polynomial. More... | |
long | getOneRow (NTL::zz_pX &row, long idx) const |
void | toPoly (NTL::ZZX &p, const IndexSet &s, bool positive=false) const |
Recovering the polynomial in coefficient representation. This yields an integer polynomial with coefficients in [-P/2,P/2], unless the positive flag is set to true, in which case we get coefficients in [0,P-1] (P is the product of all moduli used). Using the optional IndexSet param we compute the polynomial reduced modulo the product of only the primes in that set. More... | |
void | toPoly (NTL::ZZX &p, bool positive=false) const |
bool | operator== (const DoubleCRT &other) const |
bool | operator!= (const DoubleCRT &other) const |
DoubleCRT & | SetZero () |
DoubleCRT & | SetOne () |
NTL::xdouble | breakIntoDigits (std::vector< DoubleCRT > &dgts) const |
Break into n digits,according to the primeSets in context.digits. See Section 3.1.6 of the design document (re-linearization) Returns the sum of the canonical embedding of the digits. More... | |
void | addPrimes (const IndexSet &s1, NTL::ZZX *poly_p=0) |
Expand the index set by s1. It is assumed that s1 is disjoint from the current index set. If poly_p != 0, then *poly_p will first be set to the result of applying toPoly. More... | |
double | addPrimesAndScale (const IndexSet &s1) |
Expand index set by s1, and multiply by Prod_{q in s1}. s1 is disjoint from the current index set, returns log(product). More... | |
void | removePrimes (const IndexSet &s1) |
Remove s1 from the index set. More... | |
void | setPrimes (const IndexSet &s1) |
@ brief make prime set equal to s1 More... | |
const Context & | getContext () const |
const IndexMap< NTL::vec_long > & | getMap () const |
const IndexSet & | getIndexSet () const |
void | randomize (const NTL::ZZ *seed=nullptr) |
Fills each row i with random ints mod pi, uses NTL's PRG. More... | |
double | sampleSmall () |
Coefficients are -1/0/1, Prob[0]=1/2. More... | |
double | sampleSmallBounded () |
double | sampleHWt (long Hwt) |
Coefficients are -1/0/1 with pre-specified number of nonzeros. More... | |
double | sampleHWtBounded (long Hwt) |
double | sampleGaussian (double stdev=0.0) |
Coefficients are Gaussians Return a high probability bound on L-infty norm of canonical embedding. More... | |
double | sampleGaussianBounded (double stdev=0.0) |
double | sampleUniform (long B) |
Coefficients are uniform in [-B..B]. More... | |
NTL::xdouble | sampleUniform (const NTL::ZZ &B) |
void | scaleDownToSet (const IndexSet &s, long ptxtSpace, NTL::ZZX &delta) |
void | FFT (const NTL::ZZX &poly, const IndexSet &s) |
void | FFT (const zzX &poly, const IndexSet &s) |
void | reduce () const |
void | read (std::istream &str) |
void | write (std::ostream &str) const |
Arithmetic operation | |
Only the "destructive" versions are used, i.e., a += b is implemented but not a + b. | |
DoubleCRT & | Negate (const DoubleCRT &other) |
DoubleCRT & | Negate () |
DoubleCRT & | operator+= (const DoubleCRT &other) |
DoubleCRT & | operator+= (const NTL::ZZX &poly) |
DoubleCRT & | operator+= (const NTL::ZZ &num) |
DoubleCRT & | operator+= (long num) |
DoubleCRT & | operator-= (const DoubleCRT &other) |
DoubleCRT & | operator-= (const NTL::ZZX &poly) |
DoubleCRT & | operator-= (const NTL::ZZ &num) |
DoubleCRT & | operator-= (long num) |
DoubleCRT & | operator++ () |
DoubleCRT & | operator-- () |
void | operator++ (int) |
void | operator-- (int) |
DoubleCRT & | operator*= (const DoubleCRT &other) |
DoubleCRT & | operator*= (const NTL::ZZX &poly) |
DoubleCRT & | operator*= (const NTL::ZZ &num) |
DoubleCRT & | operator*= (long num) |
void | Add (const DoubleCRT &other, bool matchIndexSets=true) |
void | Sub (const DoubleCRT &other, bool matchIndexSets=true) |
void | Mul (const DoubleCRT &other, bool matchIndexSets=true) |
DoubleCRT & | operator/= (const NTL::ZZ &num) |
DoubleCRT & | operator/= (long num) |
void | Exp (long k) |
Small-exponent polynomial exponentiation. More... | |
void | automorph (long k) |
Apply the automorphism F(X) --> F(X^k) (with gcd(k,m)=1) More... | |
DoubleCRT & | operator>>= (long k) |
void | complexConj () |
Compute the complex conjugate, the same as automorph(m-1) More... | |
Friends | |
std::ostream & | operator<< (std::ostream &s, const DoubleCRT &d) |
std::istream & | operator>> (std::istream &s, DoubleCRT &d) |
Detailed Description
Implementing polynomials (elements in the ring R_Q) in double-CRT form.
Double-CRT form is a matrix of L rows and phi(m) columns. The i'th row contains the FFT of the element wrt the ith prime, i.e. the evaluations of the polynomial at the primitive mth roots of unity mod the ith prime. The polynomial thus represented is defined modulo the product of all the primes in use.
The list of primes is defined by the data member indexMap. indexMap.getIndexSet() defines the set of indices of primes associated with this DoubleCRT object: they index the primes stored in the associated Context.
Arithmetic operations are computed modulo the product of the primes in use and also modulo Phi_m(X). Arithmetic operations can only be applied to DoubleCRT objects relative to the same context, trying to add/multiply objects that have different Context objects will raise an error.
Constructor & Destructor Documentation
◆ DoubleCRT() [1/4]
|
default |
◆ DoubleCRT() [2/4]
◆ DoubleCRT() [3/4]
helib::DoubleCRT::DoubleCRT | ( | const zzX & | poly, |
const Context & | _context, | ||
const IndexSet & | indexSet | ||
) |
Same as above, but with zzX's.
◆ DoubleCRT() [4/4]
Also specify the IndexSet explicitly.
Member Function Documentation
◆ Add()
|
inline |
◆ addPrimes()
void helib::DoubleCRT::addPrimes | ( | const IndexSet & | s1, |
NTL::ZZX * | poly_p = 0 |
||
) |
Expand the index set by s1. It is assumed that s1 is disjoint from the current index set. If poly_p != 0, then *poly_p will first be set to the result of applying toPoly.
◆ addPrimesAndScale()
double helib::DoubleCRT::addPrimesAndScale | ( | const IndexSet & | s1 | ) |
Expand index set by s1, and multiply by Prod_{q in s1}. s1 is disjoint from the current index set, returns log(product).
◆ automorph()
void helib::DoubleCRT::automorph | ( | long | k | ) |
Apply the automorphism F(X) --> F(X^k) (with gcd(k,m)=1)
◆ breakIntoDigits()
NTL::xdouble helib::DoubleCRT::breakIntoDigits | ( | std::vector< DoubleCRT > & | dgts | ) | const |
Break into n digits,according to the primeSets in context.digits. See Section 3.1.6 of the design document (re-linearization) Returns the sum of the canonical embedding of the digits.
◆ complexConj()
void helib::DoubleCRT::complexConj | ( | ) |
Compute the complex conjugate, the same as automorph(m-1)
◆ Exp()
void helib::DoubleCRT::Exp | ( | long | k | ) |
Small-exponent polynomial exponentiation.
◆ FFT() [1/2]
void helib::DoubleCRT::FFT | ( | const NTL::ZZX & | poly, |
const IndexSet & | s | ||
) |
◆ FFT() [2/2]
◆ getContext()
|
inline |
◆ getIndexSet()
|
inline |
◆ getMap()
|
inline |
◆ getOneRow() [1/2]
long helib::DoubleCRT::getOneRow | ( | NTL::Vec< long > & | row, |
long | idx, | ||
bool | positive = false |
||
) | const |
Get one row of a polynomial.
◆ getOneRow() [2/2]
long helib::DoubleCRT::getOneRow | ( | NTL::zz_pX & | row, |
long | idx | ||
) | const |
◆ Mul()
|
inline |
◆ Negate() [1/2]
|
inline |
◆ Negate() [2/2]
◆ operator!=()
|
inline |
◆ operator*=() [1/4]
◆ operator*=() [2/4]
|
inline |
◆ operator*=() [3/4]
|
inline |
◆ operator*=() [4/4]
|
inline |
◆ operator++() [1/2]
|
inline |
◆ operator++() [2/2]
|
inline |
◆ operator+=() [1/4]
◆ operator+=() [2/4]
|
inline |
◆ operator+=() [3/4]
|
inline |
◆ operator+=() [4/4]
|
inline |
◆ operator--() [1/2]
|
inline |
◆ operator--() [2/2]
|
inline |
◆ operator-=() [1/4]
◆ operator-=() [2/4]
|
inline |
◆ operator-=() [3/4]
|
inline |
◆ operator-=() [4/4]
|
inline |
◆ operator/=() [1/2]
DoubleCRT & helib::DoubleCRT::operator/= | ( | const NTL::ZZ & | num | ) |
◆ operator/=() [2/2]
|
inline |
◆ operator=() [1/5]
◆ operator=() [2/5]
|
inline |
◆ operator=() [3/5]
DoubleCRT & helib::DoubleCRT::operator= | ( | const NTL::ZZ & | num | ) |
◆ operator=() [4/5]
DoubleCRT & helib::DoubleCRT::operator= | ( | const NTL::ZZX & | poly | ) |
◆ operator=() [5/5]
◆ operator==()
|
inline |
◆ operator>>=()
|
inline |
◆ randomize()
void helib::DoubleCRT::randomize | ( | const NTL::ZZ * | seed = nullptr | ) |
Fills each row i with random ints mod pi, uses NTL's PRG.
◆ read()
void helib::DoubleCRT::read | ( | std::istream & | str | ) |
◆ reduce()
|
inline |
◆ removePrimes()
|
inline |
Remove s1 from the index set.
◆ sampleGaussian()
double helib::DoubleCRT::sampleGaussian | ( | double | stdev = 0.0 | ) |
Coefficients are Gaussians Return a high probability bound on L-infty norm of canonical embedding.
◆ sampleGaussianBounded()
double helib::DoubleCRT::sampleGaussianBounded | ( | double | stdev = 0.0 | ) |
◆ sampleHWt()
double helib::DoubleCRT::sampleHWt | ( | long | Hwt | ) |
Coefficients are -1/0/1 with pre-specified number of nonzeros.
◆ sampleHWtBounded()
double helib::DoubleCRT::sampleHWtBounded | ( | long | Hwt | ) |
◆ sampleSmall()
double helib::DoubleCRT::sampleSmall | ( | ) |
Coefficients are -1/0/1, Prob[0]=1/2.
Sampling routines: Each of these return a high probability bound on L-infty norm of canonical embedding
◆ sampleSmallBounded()
double helib::DoubleCRT::sampleSmallBounded | ( | ) |
◆ sampleUniform() [1/2]
NTL::xdouble helib::DoubleCRT::sampleUniform | ( | const NTL::ZZ & | B | ) |
◆ sampleUniform() [2/2]
double helib::DoubleCRT::sampleUniform | ( | long | B | ) |
Coefficients are uniform in [-B..B].
◆ scaleDownToSet()
void helib::DoubleCRT::scaleDownToSet | ( | const IndexSet & | s, |
long | ptxtSpace, | ||
NTL::ZZX & | delta | ||
) |
◆ SetOne()
|
inline |
◆ setPrimes()
|
inline |
@ brief make prime set equal to s1
◆ SetZero()
|
inline |
◆ Sub()
|
inline |
◆ toPoly() [1/2]
void helib::DoubleCRT::toPoly | ( | NTL::ZZX & | p, |
bool | positive = false |
||
) | const |
◆ toPoly() [2/2]
void helib::DoubleCRT::toPoly | ( | NTL::ZZX & | p, |
const IndexSet & | s, | ||
bool | positive = false |
||
) | const |
Recovering the polynomial in coefficient representation. This yields an integer polynomial with coefficients in [-P/2,P/2], unless the positive flag is set to true, in which case we get coefficients in [0,P-1] (P is the product of all moduli used). Using the optional IndexSet param we compute the polynomial reduced modulo the product of only the primes in that set.
◆ write()
void helib::DoubleCRT::write | ( | std::ostream & | str | ) | const |
Friends And Related Function Documentation
◆ operator<<
|
friend |
◆ operator>>
|
friend |